railsのgeneratorを使ってファイル生成するとき、create_file以外のメソッドを覚えてますでしょうか?なかなか一つ一つのメソッドまで覚えていないと思います。今回はgeneratorの元となっているthorのモジュールを読み込んだので、全部のメソッドの使い方を紹介します。
Contents
Rails generatorで使えるファイル操作メソッド一覧
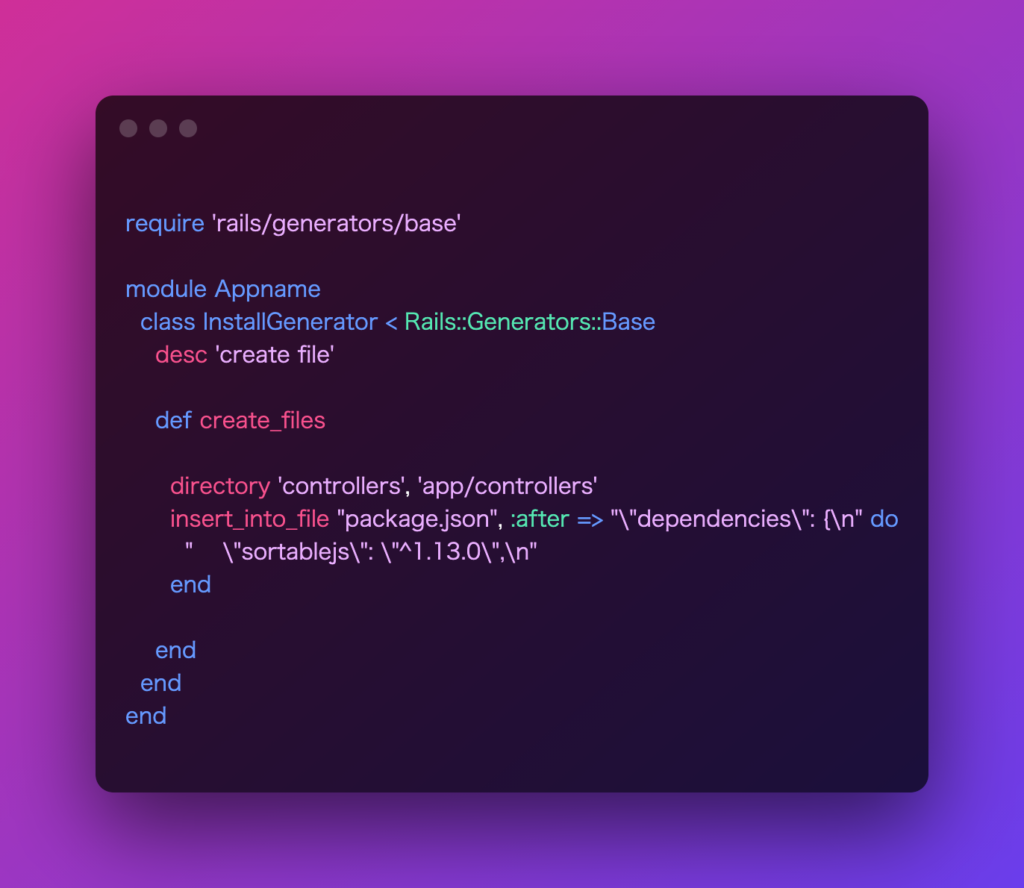
使えるファイル操作メソッドは下の19個です。
メソッド名 | 役割 |
create_file | ファイルを作成します |
add_file | 上のエイリアス |
create_link | ファイルのリンクを作成します ※1 |
add_link | 上のエイリアス |
directory | ディレクトリ配下をコピーします |
empty_directory | 空のディレクトリを作成する |
copy_file | ファイルをコピーします |
link_file | ファイルのリンクを作成します ※ |
get | ファイルの中身を取得します |
template | テンプレートファイルの内容からファイルを作成します |
chmod | ファイルの権限を変えます |
prepend_to_file | ファイルの先頭に挿入します |
prepend_file | 上のエイリアス |
append_to_file | ファイルの末尾に挿入します |
append_file | 上のエイリアス |
inject_into_class | class構文の中に挿入します |
inject_into_module | module構文の中に挿入します |
gsub_file | 正規表現に一致した文字を変更します |
uncomment_lines | 正規表現に一致した行のコメントアウトを外します |
comment_lines | 正規表現に一致した行をコメントアウトします |
remove_file | ファイルを削除します |
remove_dir | 上のエイリアス |
inject_into_file | コードを挿入します |
insert_into_file | 上のエイリアス |
※ 間違っているかも。create_linkとlink_fileの違いがわからなかったです。わかった時に修正します。
メソッドをGenerator内で使うサンプルコード
以下のように、インスタンスメソッドの中でdirectoryとかinsert_into_fileメソッドを呼び出して使います。
require 'rails/generators/base'
module Appname
class InstallGenerator < Rails::Generators::Base
desc 'create file'
def create_files
directory 'controllers', 'app/controllers'
insert_into_file "package.json", :after => "\"dependencies\": {\n" do
" \"sortablejs\": \"^1.13.0\",\n"
end
end
end
end
上の例であれば、rails generate appname:installが使えるようになるので、コマンドを入力して使います。
generatorの作り方は以下の記事で説明しているので、こちらも読んでみてください。
それぞれのメソッドの使い方
create_file
ファイルを作成します。
create_file(引数1, 引数2, 引数3)
引数1・・・作成先のパス、必須
引数2・・・オプション、任意、:verbose => true
のようにハッシュ形式で指定する。:verboseをtrueにするとログが出力される
引数3・・・挿入するデータ、必須、文字列かブロック形式で渡す
create_file "app/controllers/hello_controller.rb" do
"
class ApplicationController < ActionController::Base
end
"
end
create_file "app/controllers/hello_controller.rb", :verbose => true do
"
class ApplicationController < ActionController::Base
end
"
end
create_file "app/controllers/hello_controller.rb", "# コントローラー"
add_file
使用方法はcreate_fileと同じです。
create_link
???
多分リンクを作る
add_link
使用方法はcreate_linkと同じです。
directory
フォルダをコピーします。
directory(引数1, 引数2, 引数3)
引数1・・・元となるフォルダパス、必須
引数2・・・オプション、任意、以下を指定する
:recursive => false # 子フォルダはコピーしない
:mode => :preserve # 元のファイルの権限を保持する
:exclude_pattern => /正規表現/ # 正規表現にマッチしたファイルのみ
引数3・・・コピー先、任意、指定しなかったら元フォルダと同じフォルダパスにコピーされる
directory "models"
directory "models", :recursive => false, "app/models"
empty_directory
空のフォルダを作る。
empty_directory(引数1, 引数2)
引数1・・・フォルダのパス、必須
引数2・・・オプション、任意、:verbose => true
のようにハッシュ形式で指定する。:verboseをtrueにするとログが出力される
empty_directory "app/admins"
copy_file
執筆中
link_file
get
template
chmod
prepend_to_file
prepend_file
append_to_file
append_file
inject_into_clas
inject_into_module
gsub_file
uncomment_lines
comment_lines
remove_file
remove_dir
inject_into_file
insert_into_file
【コードリーディング】thor gem
generatorのメソッドはRailsの元となっているthorというgemで定義されています。
thorのリポジトリ
https://github.com/erikhuda/thor
generatorを作るときは以下のようにRails::Generators::BaseまたはRails::Generators::NamedBaseを継承したクラスを定義すると思います。
require 'rails/generators/base'
module Appname
class InstallGenerator < Rails::Generators::Base
desc 'create file'
def create_files
# メソッドを使用したコード
end
end
end
このRails::Generators::Baseらは内部ではthorのActionsモジュールを読み込んで作られています。
# rails/railties/lib/rails/generators/base.rb
# https://github.com/rails/rails/blob/291a3d2ef29a3842d1156ada7526f4ee60dd2b59/railties/lib/rails/generators/base.rb
省略
module Rails
module Generators
class Error < Thor::Error # :nodoc:
end
class Base < Thor::Group
include Thor::Actions
include Rails::Generators::Actions
省略
一方、Thorのgemのリポジトリを見に行くと、以下のようなディレクトリ構造になっていました。
# https://github.com/erikhuda/thor
thor
├── lib
│ ├── thor
│ │ ├── actions
│ │ │ ├── create_file.rb
│ │ │ ├── create_link.rb
│ │ │ ├── directory.rb
│ │ │ ├── empty_directory.rb
│ │ │ ├── file_manipulation.rb
│ │ │ └── inject_into_file.rb
6つのファイルがあり、それぞれの中で合計24ものメソッドが使えるようになっています。
こんな感じ
class Thor
module Actions
# Create a new file relative to the destination root with the given data,
# which is the return value of a block or a data string.
#
# ==== Parameters
# destination<String>:: the relative path to the destination root.
# data<String|NilClass>:: the data to append to the file.
# config<Hash>:: give :verbose => false to not log the status.
#
# ==== Examples
#
# create_file "lib/fun_party.rb" do
# hostname = ask("What is the virtual hostname I should use?")
# "vhost.name = #{hostname}"
# end
#
# create_file "config/apache.conf", "your apache config"
#
def create_file(destination, *args, &block)
config = args.last.is_a?(Hash) ? args.pop : {}
data = args.first
action CreateFile.new(self, destination, block || data.to_s, config)
end
alias_method :add_file, :create_file
使い方も書いてあったのでとてもわかりやすいです。
[…] Rails generatorで使えるファイル操作系メソッド24個まとめ […]